Hello world
with this plugin we are going to find all the markers in a folder and draw circles around them with user specified colors and radius.
Walkthrough
Step 1. Open Manage/Create Plugins Screen
Goto Operations & Analysis and click "Manage/Create Plugins"
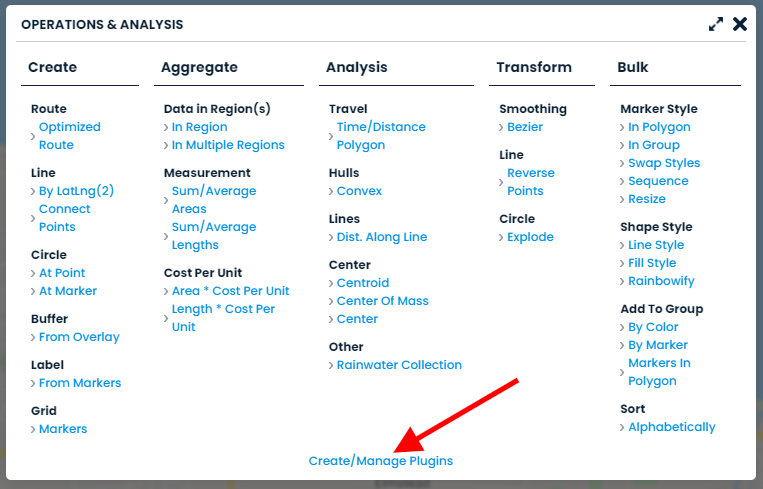
Step 2. Create New Script
Click the "Create New Script" button.
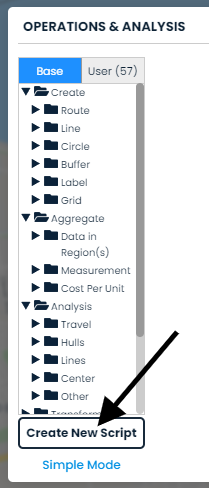
Step 3. Set Config Settings
Add the details/config JSON objects
var details = { title: "Hello World", description: "My Hello World Script", author: "Your Name" }; var config = { hasDetails: true, runLabel: "Draw Circles" //run button label };
Step 4. Set UI Elements
Next we are going to add the UI Elements we want to have in the plugin.
var ui = { 'group': { type: "group", label: "Select Group" }, 'lineColor': { type: "color", label: "Line Color" }, 'fillColor': {type: "color", label: "Fill Color" }, 'km': {type: "number", label: "Radius KM", step: 1, min: 0, max: 50, default: 5} };
We have defined four UI elements group, lineColor, fillColor, and km. These names specified will be passed in the inputs argument to the run function.
If you switch to the app view you should see the following.
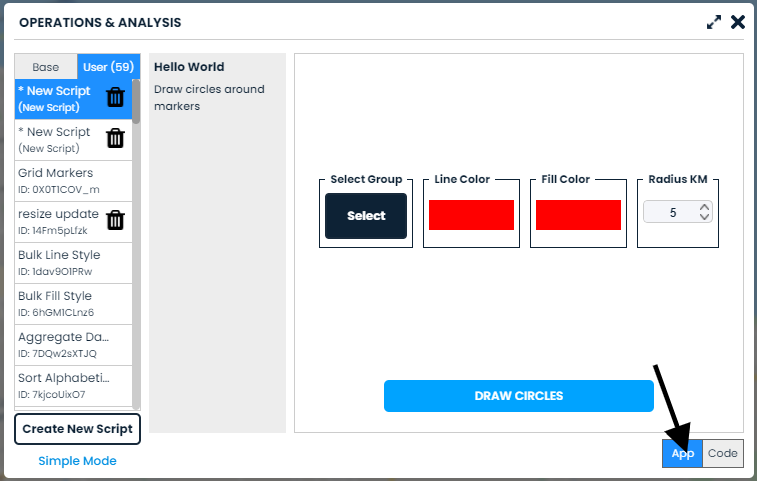
Step 5. Write Run Code
Now we can write the run code that will run when the "Draw Circles" button is pressed.
First we ar going to fetch all the overlays inside of a group specified by the user. 'group' matches what you specified in the UI config
//getGroupOverlays returns an array of overlays var overlays = api.map.getGroupOverlays(inputs.group.id);
Next, we are going to set the style so that all subsequent create circles have the style specified by the user.
api.draw.setStyle({fillColor: inputs.fillColor.color, fillOpacity: inputs.fillColor.opacity, lineColor: inputs.lineColor.color, lineOpacity: inputs.lineColor.opacity });
Now we are going to draw the circles with a radius specified by the user. Since we only want to target markers, we check the type. A marker will only ever have one coordinate so it can be reference with [0]. We use the inputs.km value specified by the user.
overlays.forEach(function(item) { if(item.type == scribblemaps.OverlayType.MARKER) { api.draw.circle(item.getCoords()[0], inputs.km + "km"); } });
Finally we want to add some feedback to the user so they know the circles have been drawn.
api.ui.showAlert("Hello World! Circles drawn!")
That's it! You are now ready to add some markers to your map in a folder to test. Here is the final run code.
var run = function (inputs, api) { var overlays = api.map.getGroupOverlays(inputs.group.id); api.draw.setStyle({fillColor: inputs.fillColor.color, fillOpacity: inputs.fillColor.opacity, lineColor: inputs.lineColor.color, lineOpacity: inputs.lineColor.opacity }); overlays.forEach(function(item) { if(item.type == scribblemaps.OverlayType.MARKER) { api.draw.circle(item.getCoords()[0], inputs.km + "km"); } }); api.ui.showAlert("Hello World! Circles drawn!") };
Step 6. Test
To test you will want to add some markers to the map in a folder.
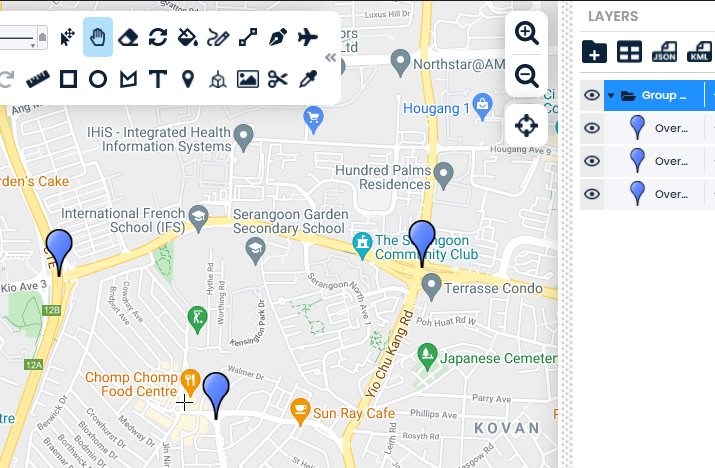
Then go back to your plugin under operations & Analysis, click "select" and select your folder.
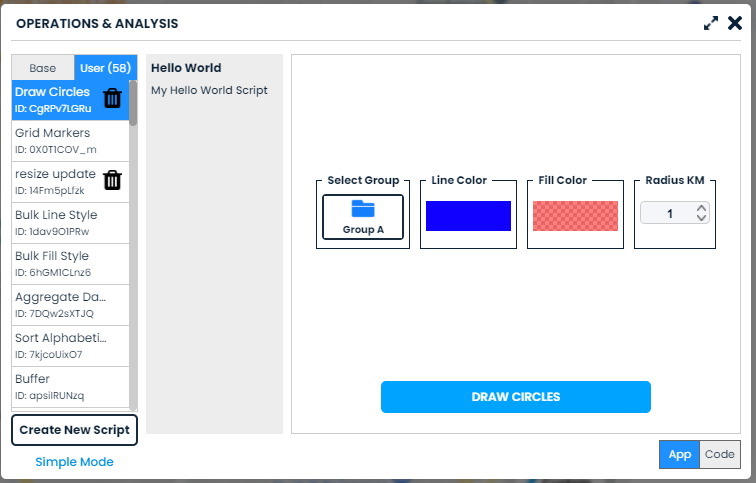
Now Click the "Draw Circles" button to run your plugin.
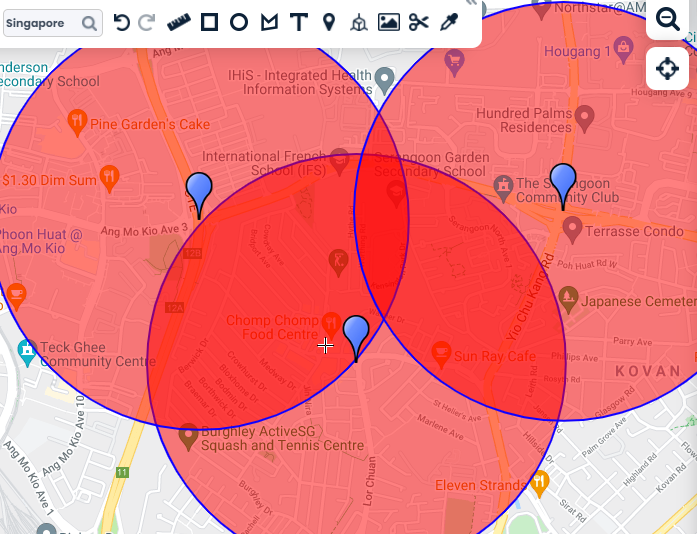
Step 7. Save
Now that you are done your plugin, you will want to save it so you can access it later. Go to the code view and click the "save" button.
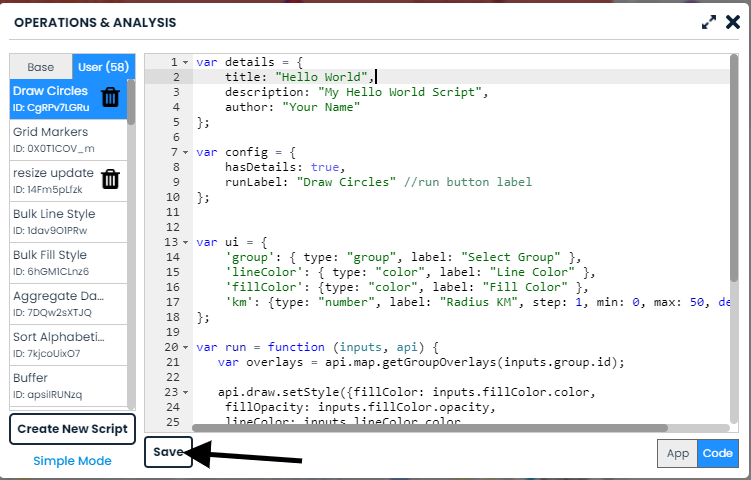
Now fill in your information and click the save button. Note the "run on startup" option to make the plugin a startup plugin.
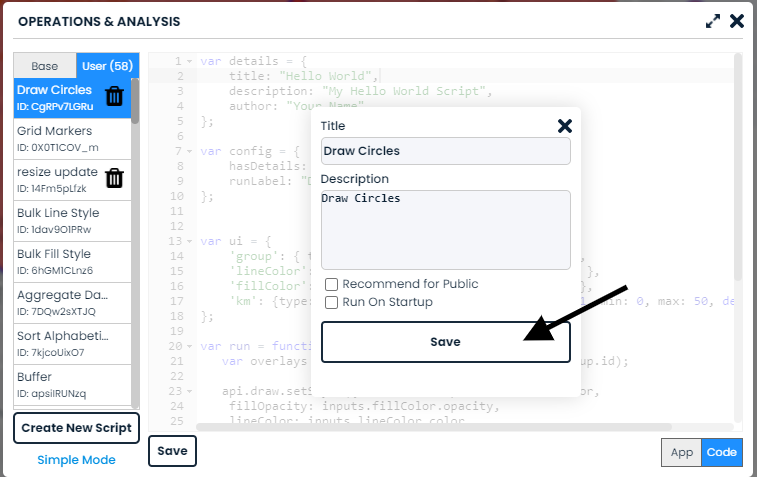
Final Code
We are all done this tutorial! Here is the final code for this plugin.
var details = { title: "Hello World", description: "My Hello World Script", author: "Your Name" }; var config = { hasDetails: true, runLabel: "Draw Circles" //run button label }; var ui = { 'group': { type: "group", label: "Select Group" }, 'lineColor': { type: "color", label: "Line Color" }, 'fillColor': {type: "color", label: "Fill Color" }, 'km': {type: "number", label: "Radius KM", step: 1, min: 0, max: 50, default: 1} }; var run = function (inputs, api) { var overlays = api.map.getGroupOverlays(inputs.group.id); api.draw.setStyle({fillColor: inputs.fillColor.color, fillOpacity: inputs.fillColor.opacity, lineColor: inputs.lineColor.color, lineOpacity: inputs.lineColor.opacity }); overlays.forEach(function(item) { if(item.type == scribblemaps.OverlayType.MARKER) { api.draw.circle(item.getCoords()[0], inputs.km + "km"); } }); api.ui.showAlert("Hello World! Circles drawn!") };